I have found vignet shader from here can we implement with cocos2dx? How?
Link : https://github.com/mattdesl/lwjgl-basics/wiki/ShaderLesson3#Step1
//Vignet.fsh
//texture 0
uniform sampler2D u_texture;
//our screen resolution, set from Java whenever the display is resized
uniform vec2 resolution;
//"in" attributes from our vertex shader
varying vec4 vColor;
varying vec2 vTexCoord;
//RADIUS of our vignette, where 0.5 results in a circle fitting the screen
const float RADIUS = 0.75;
//softness of our vignette, between 0.0 and 1.0
const float SOFTNESS = 0.45;
void main() {
//sample our texture
vec4 texColor = texture2D(u_texture, vTexCoord);
//determine center
vec2 position = (gl_FragCoord.xy / resolution.xy) - vec2(0.5);
//OPTIONAL: correct for aspect ratio
//position.x *= resolution.x / resolution.y;
//determine the vector length from center
float len = length(position);
//our vignette effect, using smoothstep
float vignette = smoothstep(RADIUS, RADIUS-SOFTNESS, len);
//apply our vignette
texColor.rgb *= vignette;
gl_FragColor = texColor;
}
//Vignet.vsh
//combined projection and view matrix
uniform mat4 u_projView;
//"in" attributes from our SpriteBatch
attribute vec2 Position;
attribute vec2 TexCoord;
attribute vec4 Color;
//"out" varyings to our fragment shader
varying vec4 vColor;
varying vec2 vTexCoord;
void main() {
vColor = Color;
vTexCoord = TexCoord;
gl_Position = u_projView * vec4(Position, 0.0, 1.0);
}
#ifdef GL_ES
precision lowp float;
#endif
varying vec4 v_fragmentColor;
varying vec2 v_texCoord;
//RADIUS of our vignette, where 0.5 results in a circle fitting the screen
//const float RADIUS = 0.75;
const float RADIUS = 0.5;
//softness of our vignette, between 0.0 and 1.0
const float SOFTNESS = 0.45;
void main() {
//sample our texture
vec4 texColor = texture2D(CC_Texture0, v_texCoord);
//determine center
//vec2 position = (gl_FragCoord.xy / resolution.xy) - vec2(0.5);
vec2 position = v_texCoord - vec2(0.5);
//OPTIONAL: correct for aspect ratio
//position.x *= resolution.x / resolution.y;
//determine the vector length from center
float len = length(position);
//our vignette effect, using smoothstep
float vignette = smoothstep(RADIUS, RADIUS-SOFTNESS, len);
//apply our vignette
texColor.rgb *= vignette;
gl_FragColor = texColor;
}
…
spr = Sprite::create("HelloWorld.png");
spr->setPosition(center);
this->addChild(spr);
std::string fragSource1 = FileUtils::getInstance()->getStringFromFile(FileUtils::getInstance()->fullPathForFilename("frag1.fsh"));
auto prog1 = GLProgram::createWithByteArrays(ccPositionTextureColor_noMVP_vert, fragSource1.data());
auto programState1 = GLProgramState::getOrCreateWithGLProgram(prog1);
spr->setGLProgramState(programState1);
result:
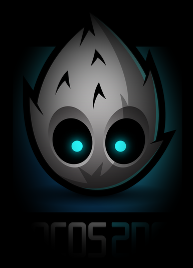
2 Likes
Thanks a lot. I knew only you can do this. 
same way i modify working color correction shader for whole game scene. by that way last time also you have implemented ccTexture0 to bind texture to the shader. what is it by the way?
Is this below code required to use :
#ifdef GL_ES
precision lowp float;
#endif
in every sahder.fsh file? like we use for c++?